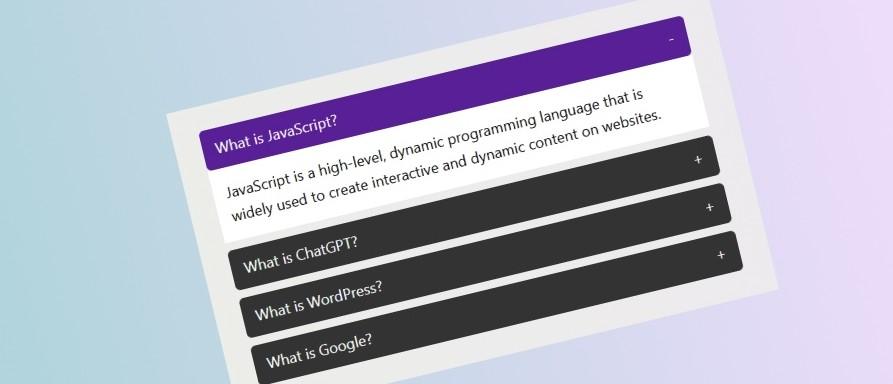
You will find accordion contents on many modern websites. An accordion is a UI pattern where content is toggled between expanded and collapsed states. This allows web developer to show more information in a small area and in a modern style. Accordions are great for enhancing the usability of your site by saving space and keeping your content organized.
In this blog post, we’ll explore how to build a simple accordion using HTML, CSS, and JavaScript. Let’s dive right in!
HTML Structure
<div class="accordion-items">
<div class="accordion-item">
<div class="accordion-title">What is JavaScript?</div>
<div class="accordion-body">
<p>JavaScript is a high-level, dynamic programming language that is widely used to create interactive and dynamic content on websites.</p>
</div>
</div><!--/accordion-item-->
<div class="accordion-item">
<div class="accordion-title">What is ChatGPT?</div>
<div class="accordion-body">
<p>ChatGPT is an advanced AI language model developed by OpenAI. It is based on the GPT to understand and generate human-like text.</p>
<p>However, you can use composer to manage your website.</p>
</div>
</div><!--/accordion-item-->
<div class="accordion-item">
<div class="accordion-title">What is WordPress?</div>
<div class="accordion-body">
<p>WordPress is a popular open-source content management system (CMS) used to create, manage, and publish websites.</p>
</div>
</div><!--/accordion-item-->
<div class="accordion-item">
<div class="accordion-title">What is Google?</div>
<div class="accordion-body">
<p>Google is a global technology company best known for its search engine, which is one of the most widely used tools for finding information on the internet.</p>
</div>
</div><!--/accordion-item-->
</div><!--/accordion-items-->
CSS for Styling
Let’s add some simple styles to make the accordion look good and ensure it behaves correctly.
/* Accordion */
.accordion-items {
display: flex;
flex-direction: column;
gap: 10px;
}
.accordion-item {
display: flex;
flex-direction: column;
}
.accordion-title {
position: relative;
background-color: #333333;
color: #ffffff;
padding: 10px 16px;
border-radius: 6px;
cursor: pointer;
transition: background-color 0.3s linear;
}
.accordion-title::after {
position: absolute;
content: "+";
right: 16px;
}
.accordion-body {
background-color: #ffffff;
padding: 0 16px;
height: 0px;
overflow: hidden;
transition: all 0.3s linear;
}
.accordion-body p:last-of-type {
margin: 0;
}
.accordion-active {
background-color: #581F96;
}
.accordion-active::after {
content: "-";
}
.accordion-active + .accordion-body {
height: unset;
padding: 16px;
}
JavaScript for Toggle Functionality
We will use JavaScript to toggle the content sections when the user clicks the accordion title.
Here’s the JavaScript code:
const accItem = document.getElementsByClassName('accordion-title');
let i;
for (i = 0; i < accItem.length; i++) {
accItem[i].addEventListener("click", function(){
this.classList.toggle("accordion-active");
});
}
In this tutorial, we have built a simple accordion component from scratch using HTML, CSS, and JavaScript. The accordion we created allows users to expand and collapse sections of content by clicking the title of section.
Feel free to modify this accordion with additional features, such as color, animations, icons. Happy coding!
- Log in to post comments