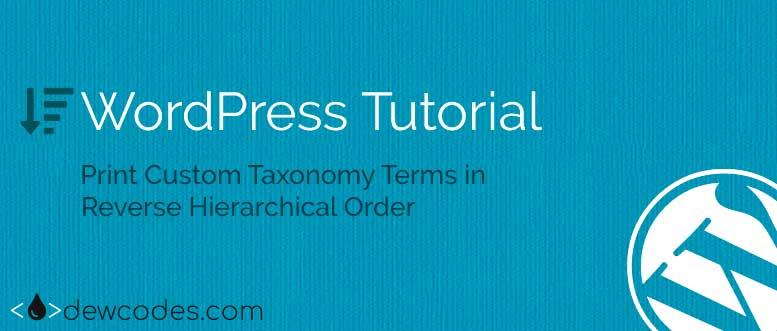
In this WordPress tutorial we will learn how to print / display terms of a custom taxonomy or WordPress default category in hierarchical order and reverse hierarchical order in single post.
Parent
– child
— sub-child
WordPress Default Display
By default, WordPress will print these taxonomy terms in alphabetical order.
But we want to display these terms in hierarchical order and reverse hierarchical order.
Display Terms in Hierarchical Order
To display terms of a custom taxonomy or terms of WordPress default category in hierarchical order in single post like below:
Parent, child, sub-child
Use below code in single.php where you want to print the taxonomy terms.
<?php
/*
* Print Taxonomy Terms in Hierarchical Order
*/
$taxonomy = 'YOUR_TAXONOMY_NAME'; // change this to your custom taxonomy name.
$taxonomy_terms = wp_get_post_terms( $post->ID, $taxonomy, array( "fields" => "ids" ) );
if( $taxonomy_terms ) {
$term_array = trim( implode( ',', (array) $taxonomy_terms ), ' ,' );
$neworderterms = get_terms($taxonomy, 'orderby=none&include=' . $term_array );
foreach( $neworderterms as $orderterm ) {
echo '' . $orderterm->name . ', ';
}
}
?>
Note: Change YOUR_TAXONOMY_NAME to your taxonomy name.
Print Taxonomy Terms in Reverse Hierarchical Order
If you requirement is to print taxonomy terms in reverse hierarchical order like:
Sub-child, Child, Parent
Then use below code in single.php file.
<?php
/*
* Print Taxonomy Terms in Reverse Hierarchical Order
*/
$taxonomy = 'YOUR_TAXONOMY_NAME'; // change this to your custom taxonomy name.
$taxonomy_terms = wp_get_post_terms( $post->ID, $taxonomy, array( "fields" => "ids" ) );
if( $taxonomy_terms ) {
$term_array = trim( implode( ',', (array) $taxonomy_terms ), ' ,' );
$neworderterms = get_terms($taxonomy, 'orderby=none&include=' . $term_array );
foreach( array_reverse($neworderterms) as $orderterm ) {
echo '' . $orderterm->name . ', ';
}
}
?>
Note: Change YOUR_TAXONOMY_NAME to your taxonomy name.
- Log in to post comments