26 Dec 2023
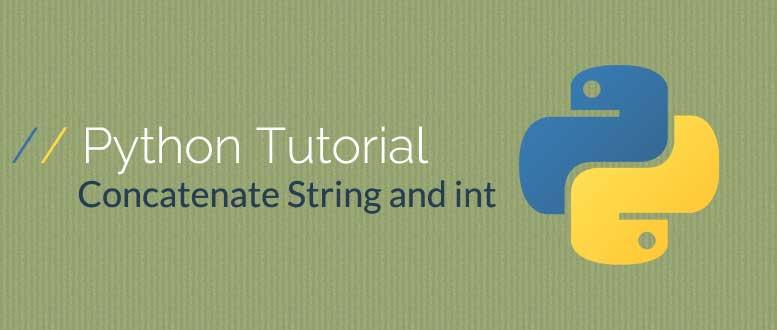
Concatenation Operator in Python
In Python + is used as concatenation operator. By default, Python supports string concatenation using + operator. And if you try to concatenate string and integer using + operator, it will throw some runtime error.
Example
Let's try to concatenate string and integer using + operator.
message = "Hello and welcome to GelCode "
number = 100
print(message + number)
Result
print(message + number)
TypeError: can only concatenate str (not "int") to str
Process finished with exit code 1
So how to concatenate string and integer in Python? There are various ways to do this, below I have listed few methods.
Method 1: Using str() function
Convert the integer to string type in print function using str() function.
message = "Hello and welcome to GelCode "
num = 100
print(message + str(num))
Output
Hello and welcome to GelCode 100
Method 2: Using f-strings
message = "Hello and welcome to GelCode "
number = 100
print(f'{message}{number}')
Output
Hello and welcome to GelCode 100
You can also use string directly with f-strings. See below example.
brand = "GelCode "
number = 100
print(f'{"Hello and welcome to "}{brand}{number}')
Output
Hello and welcome to GelCode 100
- Log in to post comments