26 Dec 2023
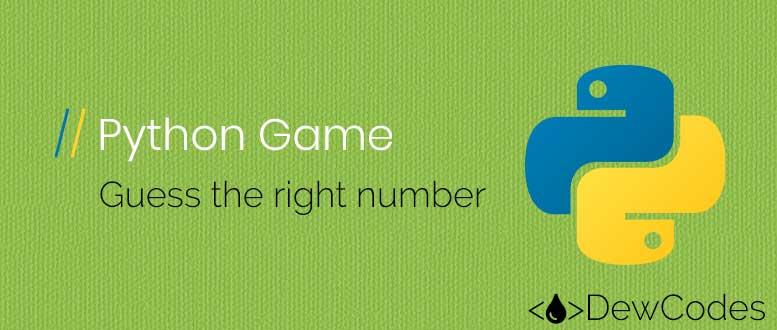
In this python tutorial, we will create a small game. User will be asked to guess a single digit number (0-9). User will be allowed to guess maximum 3 times.
If the guessed number is correct, it will show congratulation message. If user failed to guess the right number in maximum 3 tries, he will get message that he has guessed maximum times and will also show the right number.
Every time, the game is played, it will generate a random correct number.
So, here is the complete code.
# import random module
import random
right_number = random.randint(0,9)
guess_limit = 3
guess_count = 1
while guess_count <= guess_limit:
guess_number = int(input("Guess the right number: "))
if (guess_number == right_number ):
print("Congratulations!!! You have guessed the right number")
break
else:
print("Sorry wrong number. Please try again!!")
guess_count = guess_count + 1
else:
print(f"Sorry, You have guesses maximum allowed times. The correct number is {right_number}")
- Log in to post comments